Motivation ^_^
I know learning a new thing needs the answer to “WHY SHOULD I EVEN LEARN THIS STUFF? HOW CAN I APPLY THIS LEARNING IN PRACTICAL LIFE?”. So here we go for the answer now..
Python is necessary not only for software devs but also for an accountant, an economic expert, a marketer, a finance expert, a genetic engineer and also for higher study enthusiasts.
A common question of newbie programmers these days is why should I learn python first instead of C/C++? The simple answer starts with a simple question “Why Shouldn’t You?”
Python is easy to learn compared to C, C++ or java. It is one of the top programming languages of 2021.
Python has a wide range of libraries which are used in big projects. These library functions are customizable.
Python is an open source language meaning you can develop library functions of python yourself.
Python supports cross platform development. What is cross platform development? If you develop an android application it would work in IOS as well. Even if you develop a desktop application it can be executed in any operating systems WINDOWS or LINUX
Python has two very famous frameworks for backend development. Flask and Django (read it jango). So for job preparation one can prepare him/herself learning any of the two frameworks very well.
In higher studies , in different sectors python is needed badly. Such as Artificial Intelligence, Machine learning, Data Science.
The job sector for python is huge. You can be a web dev, software dev, data scientist, business analyst, ML engineer, game dev, network programmer and what not.
So if you excel in only one programming language python, then your career will have the boost it needs for sure <3.
Day 3 Basics
Object Oriented Programming
Object:
An object is simply a collection of data (variables) and methods (functions) that act on those data. A parrot is an object, as it has the following properties: name, age, color as attributes and singing, dancing as behavior. An object is also called an instance of a class and the process of creating this object is called instantiation. The concept of OOP in Python focuses on creating reusable code. This concept is also known as DRY (Don't Repeat Yourself).
Class:
A class is a blueprint for the object. We can think of class as a sketch of a parrot with labels. It contains all the details about the name, colors, size etc. Based on these descriptions, we can study about the parrot. Here, a parrot is an object. From class, we construct instances. An instance is a specific object created from a particular class. When class is defined, only the description for the object is defined. Therefore, no memory or storage is allocated.
Methods:
Methods are functions defined inside the body of a class. They are used to define the behaviors of an object.
Inheritance:
Inheritance is a way of creating a new class for using details of an existing class without modifying it. The newly formed class is a derived class (or child class). Similarly, the existing class is a base class (or parent class).
class BaseClass: Body of base class class DerivedClass(BaseClass): Body of derived class
Method Overriding
Generally when overriding a base method by a derived method, we tend to extend the definition of base method rather than replacing it.
Multiple Inheritance
A class can be derived from more than one base class in Python, similar to C++. This is called multiple inheritance. In multiple inheritance, the features of all the base classes are inherited into the derived class.
class Base1: pass class Base2: pass class MultiDerived(Base1, Base2): pass
Multilevel Inheritance
We can also inherit from a derived class. This is called multilevel inheritance. It can be of any depth in Python. In multilevel inheritance, features of the base class and the derived class are inherited into the new derived class.
class Base: pass class Derived1(Base): pass class Derived2(Derived1): pass
Encapsulation
Using OOP in Python, we can restrict access to methods and variables. This prevents data from direct modification which is called encapsulation. In Python, we denote private attributes using underscore as the prefix i.e single _ or double __.
Polymorphism
Polymorphism allows the same interface for different objects. Suppose, we need to color a shape, there are multiple shape options (rectangle, square, circle). However we could use the same method to color any shape. This concept is called Polymorphism.
Dictionary
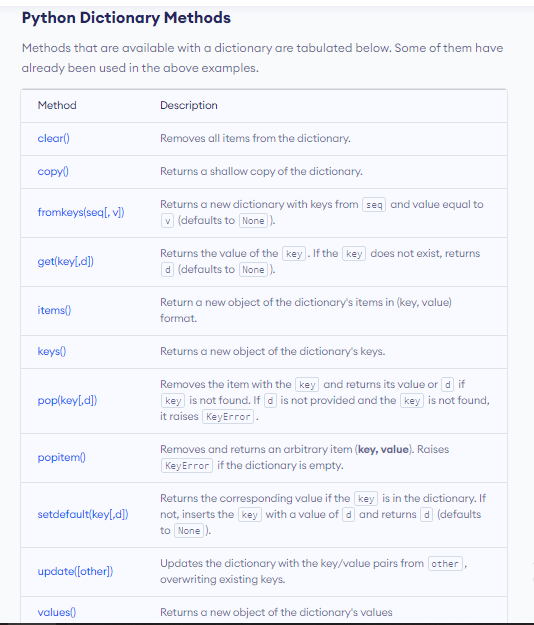Lists
- [list_basics](https://github.com/Geek-a-Byte/PyHaxx/blob/main/list.py) - [list_comprehension](https://github.com/Geek-a-Byte/PyHaxx/blob/main/List_comprehension.py) 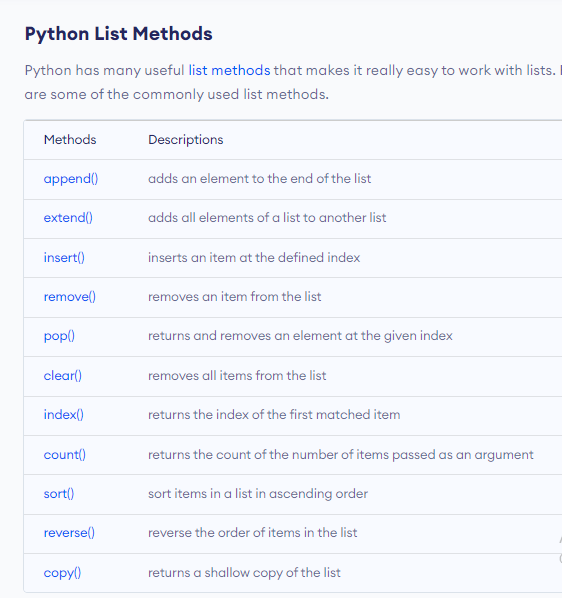Working with external libraries
[tutorial link](https://app.gitbook.com/s/98WyytUmB4pfdMH3QiQy/)Exceptions
We can make certain mistakes while writing a program that lead to errors when we try to run it. A python program terminates as soon as it encounters an unhandled error. These errors can be broadly classified into two classes: - Syntax errors - Logical errors (Exceptions)
Python Syntax Errors
Error caused by not following the proper structure (syntax) of the language is called syntax error or parsing error.
Python Logical Errors (Exceptions)
Errors that occur at runtime (after passing the syntax test) are called exceptions or logical errors. For instance, - they occur when we try to open a file(for reading) that does not exist (FileNotFoundError) - try to divide a number by zero (ZeroDivisionError) - try to import a module that does not exist (ImportError)
Whenever these types of runtime errors occur, Python creates an exception object. If not handled properly, it prints a traceback to that error along with some details about why that error occurred. When these exceptions occur, the Python interpreter stops the current process and passes it to the calling process until it is handled. If not handled, the program will crash.
Python Built-in Exceptions
Illegal operations can raise exceptions. There are plenty of built-in exceptions in Python that are raised when corresponding errors occur. We can view all the built-in exceptions using the built-in local() function as follows:
print[dir(locals(]('__builtins__')))
Catching Exceptions in Python
In Python, exceptions can be handled using a try statement. The critical operation which can raise an exception is placed inside the try clause. The code that handles the exceptions is written in the except clause.
User Defined Exceptions
[Learn how to define an exception](https://www.programiz.com/python-programming/user-defined-exception)Map
map() Parameter
The map() function takes two parameters: function - a function that perform some action to each element of an iterable iterable - an iterable like sets, lists, tuples, etc You can pass more than one iterable to the map() function.
Map Return Value
The map() function returns an object of map class. The returned value can be passed to functions like list() - to convert to list set() - to convert to a set, and so on.
Filter
The filter() function extracts elements from an iterable (list, tuple etc.) for which a function returns True.
filter() Syntax
filter(function, iterable)
filter() Parameter
function - a function that perform some action to each element of an iterable iterable - an iterable like sets, lists, tuples, etc
Return Value
The filter() function returns an iterator. You can easily convert iterators to sequences like lists, tuples, strings etc.
Lambda Functions
an anonymous function is a function that is defined without a name. While normal functions are defined using the def keyword in Python, anonymous functions are defined using the lambda keyword. Hence, anonymous functions are also called lambda functions.
Syntax of Lambda Function in python
lambda arguments: expression
Resources
- Programiz (the easiest explanation so far according to me)
- Tamim Subeen’s pybook
- Geeksforgeeks
- Real Python
- Python 3.10.0 tutorial documentation
- Kaggle
- Playlist of mahmudul haque bhaiya for MIC python course(last year)
- freecodecamp
- Sumon’s Acamedy
- Udemy